Why is Rust Revolutionizing Systems Programming?
Explore how Rust is transforming low-level programming with its revolutionary approach to memory safety and zero-cost abstractions. Learn why major tech companies are adopting Rust and how it combines C++'s performance with modern development features.
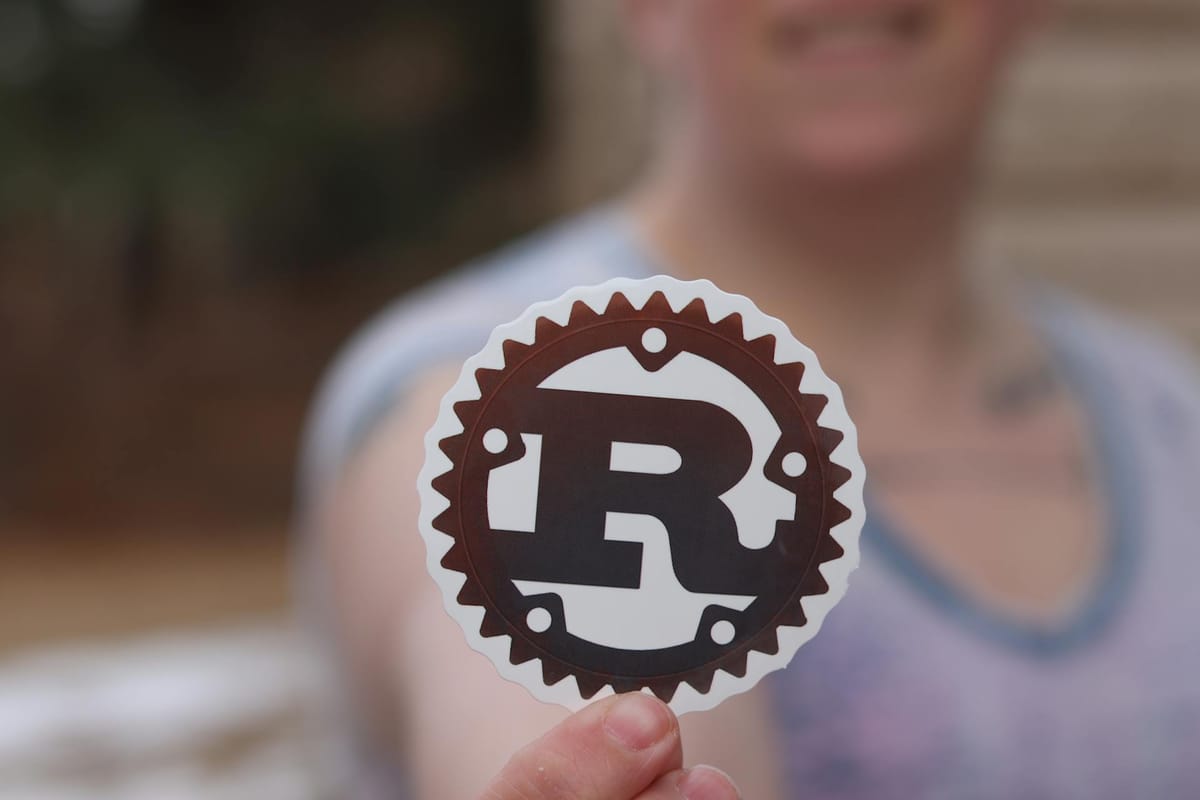
Let me merge these posts while preserving the unique insights from both and maintaining a cohesive flow.
A Deep Dive into Safety, Performance, and Modern Development
C and C++ have traditionally dominated system programming. While these languages offer exceptional performance, they often require developers to make challenging tradeoffs between safety, maintainability, and development speed. Rust, introduced in 2010, is fundamentally transforming this landscape by proving that systems programming can be safe, concurrent, and fast without a compromise.
The Historical Context
Memory-related bugs have plagued system programming for decades. The Computing Research Association estimates that approximately 70% of serious security vulnerabilities stem from memory management issues. This historical context makes Rust's innovations particularly significant.
Memory Safety: Rust's Game-Changing Innovation
Rust's most groundbreaking feature is its ability to ensure memory safety without relying on a garbage collector. Through its ownership system, Rust enforces strict rules about memory allocation and access at compile time:
fn demonstrate_ownership() {
let s1 = String::from("Hello, Rust!");
let s2 = s1; // Ownership moves to s2
// println!("{}", s1); // This would cause a compile error
let s3 = String::from("Hello, Borrowing!");
let s4 = &s3; // Borrowing s3's value
println!("Original: {}, Borrowed: {}", s3, s4); // Both valid
}
This ownership model prevents:
- Null pointer dereferences
- Buffer overflows
- Data races
- Use-after-free errors
- Double-free errors
Performance That Rivals C and C++
Rust achieves performance comparable to C and C++ through zero-cost abstractions. These allow developers to write high-level, expressive code that compiles down to efficient machine code:
fn demonstrate_zero_cost() {
// High-level, iterator-based approach
let numbers = vec![1, 2, 3, 4, 5];
let squares: Vec<i32> = numbers.iter()
.map(|x| x * x)
.collect();
// Compiles to code as efficient as manual iteration
println!("Squared values: {:?}", squares);
}
Concurrency Without Fear
Modern software increasingly relies on concurrent programming. Rust makes this safer by enforcing thread safety at compile time:
use std::thread;
use std::sync::{Arc, Mutex};
fn demonstrate_safe_concurrency() {
let counter = Arc::new(Mutex::new(0));
let mut handles = vec![];
// Spawn multiple threads safely
for i in 0..5 {
let counter_clone = Arc::clone(&counter);
let handle = thread::spawn(move || {
let mut num = counter_clone.lock().unwrap();
*num += 1;
println!("Thread {} modified counter", i);
});
handles.push(handle);
}
// Wait for all threads to complete
for handle in handles {
handle.join().unwrap();
}
}
A Mature and Growing Ecosystem
Rust's ecosystem has evolved significantly, offering:
- Cargo: A sophisticated package manager that handles dependencies and builds
- Tokio: A powerful async runtime for building concurrent applications
- WebAssembly Support: First-class support for compiling to WASM
- Rich Standard Library: Comprehensive built-in functionality
Example of modern async Rust using Tokio:
use tokio::time::{sleep, Duration};
#[tokio::main]
async fn main() {
println!("Starting async operation...");
sleep(Duration::from_secs(2)).await;
println!("Completed after 2 seconds");
}
Industry Adoption and Real-World Impact
Major technology companies are increasingly adopting Rust for critical systems:
- AWS: Uses Rust for Firecracker, powering Lambda and other serverless offerings
- Microsoft: Exploring Rust for Windows components and Azure IoT Edge
- Google: Implementing Rust in Android's system components
- Meta: Adopting Rust for source control backend
- Discord: Rewrote their game SDK in Rust, achieving 50% memory reduction
- Cloudflare: Implementing WASM modules and security-critical elements
Forward-Thinking Language Design
Rust's design philosophy emphasizes catching errors at compile time through features like exhaustive pattern matching:
enum ConnectionState {
Connected(String),
Disconnected,
Reconnecting(u32)
}
fn handle_connection(state: ConnectionState) {
match state {
ConnectionState::Connected(addr) => println!("Connected to {}", addr),
ConnectionState::Disconnected => println!("No connection"),
ConnectionState::Reconnecting(attempt) => println!("Attempt {}", attempt),
// Compiler ensures we handle all cases
}
}
Looking to the Future
As software systems become more complex and security becomes increasingly critical, Rust's guarantees become more valuable. The language is especially well-positioned for:
- Operating Systems Development
- Embedded Systems
- WebAssembly Applications
- Cloud Infrastructure
- Game Development
- Security-Critical Applications
Conclusion
Rust represents a fundamental shift in how we approach systems programming. By combining safety, performance, and modern programming concepts, it offers a compelling vision for the future of low-level software development. As security and reliability become increasingly crucial, Rust's compile-time guarantees and zero-cost abstractions make it an ideal choice for modern system programming.
Whether you're building operating systems, embedded devices, or cloud infrastructure, Rust provides the tools and safeguards needed for robust, maintainable, and efficient software development.
What are your thoughts on Rust's role in the future of systems programming? Share your experiences and join the discussion in the comments below!
Comments ()