C# 13: Key Features You Need to Know About
C# 13 introduces new features like advanced params usage, better thread synchronization, and optimizations for method resolution. It also supports ref struct in async methods and enhances safety, performance, and readability, empowering developers to write cleaner, more efficient code.
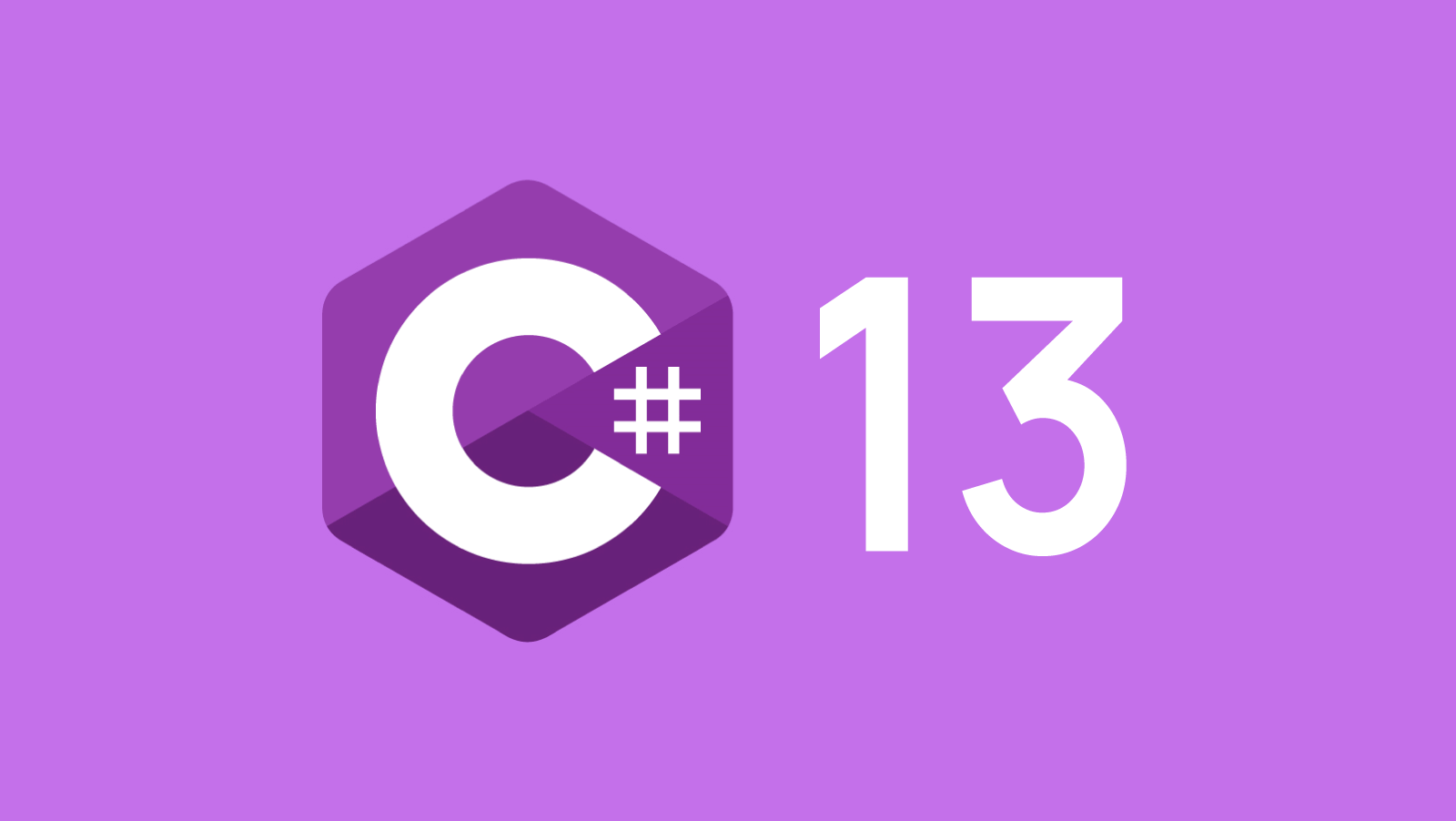
C# 13: Key Features You Need to Know About
C# 13 brings a host of new features that enhance flexibility, improve performance, and simplify syntax for developers. Here’s a detailed look at some of the most notable updates.
1. Enhanced params
Modifier
The params
modifier is no longer limited to arrays. You can now use it with types such as System.Span<T>
, System.ReadOnlySpan<T>
, and collections implementing IEnumerable<T>
with an Add
method. This allows for more versatile method signatures and reduces boilerplate code.
public void Concat<T>(params ReadOnlySpan<T> items)
{
foreach (var item in items)
{
Console.Write(item + " ");
}
Console.WriteLine();
}
This feature enables a clean approach to passing multiple collections into a method.
2. New Lock
Object for Thread Synchronization
C# 13 introduces a new synchronization primitive: the Lock
object. This is a modern replacement for Monitor.Enter
/Exit
and provides a more intuitive API. With the Lock.EnterScope()
method, you can enter an exclusive scope, and the returned ref struct ensures that the scope is exited correctly via the Dispose()
pattern.
Lock myLock = new Lock();
myLock.EnterScope();
try
{
// thread-safe operations
}
finally
{
myLock.Dispose();
}
This eliminates common pitfalls associated with traditional locking mechanisms.
3. Method Group Optimizations
Method group resolution has been optimized to make overload choice more efficient. Previously, the compiler would check all overloads for a method group, even those that were not applicable. With C# 13, the compiler prunes irrelevant methods early, leading to faster compilation times and less ambiguity in overload resolution.
public void PrintMessage() => Console.WriteLine("Hello, C# 13!");
Action printAction = PrintMessage;
In this example, only the applicable overload of PrintMessage
is considered, optimizing the resolution process.
4. New Escape Sequence: \e
In C# 13, a new escape sequence, \e
, has been introduced to represent the ESCAPE character (Unicode U+001B). This simplifies the usage of escape sequences, removing the ambiguity that existed when using hexadecimal notation (\x1b
).
Console.WriteLine("This is the escape character: \e");
This improvement makes string manipulation involving control characters more readable.
5. Implicit Index Access in Object Initializers
C# 13 now allows using the ^
operator for indexing from the end of collections in object initializers, making initialization cleaner and more intuitive.
var countdown = new TimerRemaining()
{
buffer =
{
[^1] = 0,
[^2] = 1,
[^3] = 2
}
};
This creates a countdown array, and the use of ^
allows for backward indexing directly in the object initializer.
6. Support for ref
in Async Methods and Iterators
Previously, ref
locals and unsafe
contexts weren’t allowed in async methods or iterator methods (using yield return
). With C# 13, you can now safely use ref
types and unsafe
code in these contexts, enabling more efficient memory management and better performance with types like Span<T>
and ReadOnlySpan<T>
.
public async Task ProcessDataAsync()
{
ref int data = ref myData[0];
await Task.Delay(1000);
}
This feature makes working with large data more efficient by minimizing unnecessary allocations.
7. allows ref struct
Anti-Constraint for Generics
C# 13 introduces the ability to use ref struct
types as generic type parameters. This opens up possibilities for creating high-performance, memory-safe algorithms that can still utilize ref struct
types like Span<T>
in generic contexts.
public class Container<T> where T : allows ref struct
{
public void AddData(scoped T item)
{
// safely use ref structs
}
}
This allows you to pass ref struct
types as type parameters while maintaining the compiler’s strict safety checks.
8. ref struct
Types Can Now Implement Interfaces
Another significant change in C# 13 is that ref struct
types can now implement interfaces. This allows for more flexible and extensible designs without compromising the safety of ref struct
types.
public ref struct MyStruct : IPrintable
{
public void Print() => Console.WriteLine("Printing MyStruct");
}
Now ref struct
types like MyStruct
can implement interfaces such as IPrintable
.
9. More Partial Members
C# 13 extends support for partial members, allowing you to declare partial properties and partial indexers. This gives you more control over how your properties and indexers are implemented without modifying the original class structure.
public partial class MyClass
{
// Declaring declaration
public partial string Name { get; set; }
}
public partial class MyClass
{
// Implementation declaration
private string _name;
public partial string Name
{
get => _name;
set => _name = value;
}
}
This feature supports better separation of concerns, especially in generated code.
10. Overload Resolution Priority
The new OverloadResolutionPriorityAttribute
allows library authors to control which overload is preferred by the compiler. This ensures that newer, more efficient overloads are chosen over older ones without breaking existing code.
[OverloadResolutionPriority(1)]
public void PerformAction(string name) { /* ... */ }
This helps to resolve ambiguity when adding new overloads, improving performance without causing compatibility issues.
11. Preview Feature: The field
Keyword
The new field
keyword, available as a preview feature, lets you access a compiler-synthesized backing field in a property accessor. This eliminates the need to manually declare a backing field in certain cases, simplifying your code.
public string Name { get => field; set => field = value; }
You must enable this feature by setting <LangVersion>Preview</LangVersion>
in your project file. However, care should be taken when using it, as it may cause ambiguity if a field is also named field
.
Conclusion
C# 13 introduces several important features that aim to make the language more powerful, efficient, and developer-friendly. From improvements to params
, the new Lock
synchronization object, and better support for ref struct
types, C# 13 offers many enhancements for both everyday development and high-performance scenarios. Keep an eye on the official documentation and feedback channels to make the most of these new capabilities in your projects.
Comments ()